Spinner from PHP and MySQL using JSON
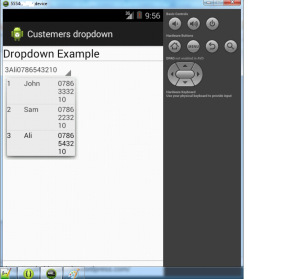
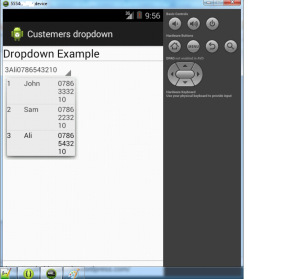
***Pertama buatlah database seperti ini :
CREATE TABLE `customer` (
`customerID` int(2) NOT NULL,
`name` varchar(50) NOT NULL,
`phone` varchar(50) NOT NULL,
PRIMARY KEY (`customerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `customer`
--
INSERT INTO `customer` VALUES (1, 'John', '0786333210');
INSERT INTO `customer` VALUES (2, 'Sam', '0786223210');
INSERT INTO `customer` VALUES (3, 'Ali', '0786543210')
***Kedua buatlah koneksi dengan Script PHP seperti ini :
<?php
$objConnect = mysql_connect("localhost","YourName","YourPassword");
$objDB = mysql_select_db("customers");
$strSQL = "SELECT * FROM customer WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
Berinama getCustomers.php dan simpan di polder C://XAMPP/htdocs.
***Ketiga buatlah project di eclipse baru dengan ketentuan sgi brkut :
Application Name : “Custemers dropdown”
Project Name : CustemersDropdown
Package Name : com.custemersdropdown
***Kempat rubahlah isi dari AndroidManifest.xml dengan kode di bawah ini :
<uses-permission android:name="android.permission.INTERNET"/>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.custemersdropdown"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="18" />
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.custemersdropdown.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
***Klima isikan printah di bawah ini pada activity_main.xml :
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Dropdown Example "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="https://androidappcode.wordpress.com/" />
</LinearLayout>
</TableLayout>
***Kenam buatlah Layout baru dengan nama activity_show.xml dan isikan perintah di bawah ini :
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColCustomerID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="CustomerID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
***Ketujuh isikan/copy'kan perintah di bawah ini pada class MainActivity.java :
package com.custemersdropdown;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.SimpleAdapter;
import android.widget.Spinner;
import android.widget.Toast;
@SuppressLint("NewApi") public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// spinner1
final Spinner spin = (Spinner)findViewById(R.id.spinner1);
String url = "http://10.0.2.2/customer/getcustomers.php";
try {
JSONArray data = new JSONArray(getJSONUrl(url));
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("customerID", c.getString("customerID"));
map.put("name", c.getString("name"));
map.put("phone", c.getString("phone"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_show,
new String[] {"customerID", "name", "phone"}, new int[] {R.id.ColCustomerID, R.id.ColName, R.id.ColTel});
spin.setAdapter(sAdap);
final AlertDialog.Builder viewDetail = new AlertDialog.Builder(this);
spin.setOnItemSelectedListener(new OnItemSelectedListener() {
public void onItemSelected(AdapterView<?> arg0, View selectedItemView,
int position, long id) {
String sCustomerID = MyArrList.get(position).get("customerID")
.toString();
String sName = MyArrList.get(position).get("name")
.toString();
String sTel = MyArrList.get(position).get("phone")
.toString();
viewDetail.setIcon(android.R.drawable.btn_star_big_on);
viewDetail.setTitle("Customer Detail");
viewDetail.setMessage("customerID : " + sCustomerID + "\n"
+ "name : " + sName + "\n" + "Tel : " + sTel);
viewDetail.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
// TODO Auto-generated method stub
dialog.dismiss();
}
});
viewDetail.show();
}
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this,
"Your Selected : Nothing",
Toast.LENGTH_SHORT).show();
}
});
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
***Terakhir Run lah program anda makan akan jadi seperti ini :
Ok thank,,,jika ada pertanyaan mengenai tutorial di atas silahkan berkomentar..!!!!
0 comments:
Post a Comment